The Ultimate Guide to Frontend Testing
Testing is a really important part of development processes, especially as frontend engineers. Frontend applications are often complex and interactive, and they can be difficult to test manually. Testing frameworks can help frontend engineers to automate their tests and to catch bugs early on before they cause problems for users.
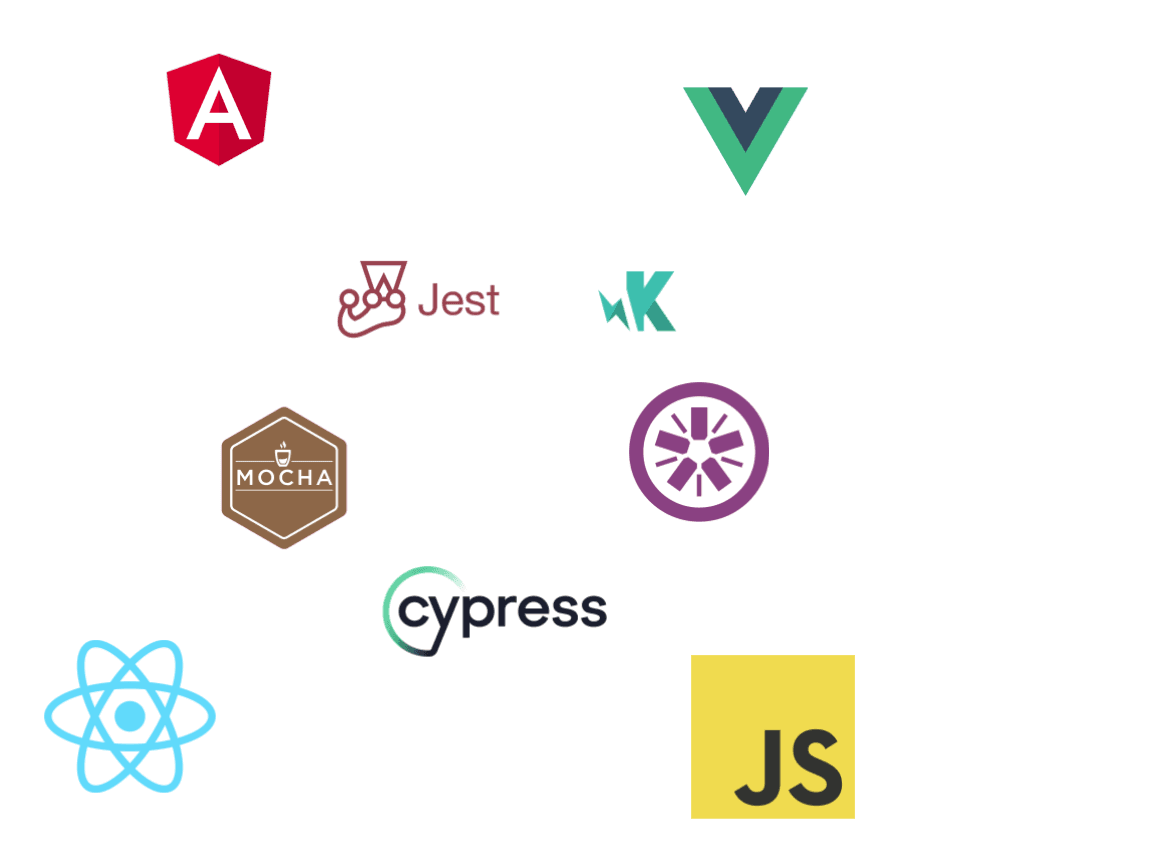
Testing is a really important part of development processes, especially as frontend engineers. Frontend applications are often complex and interactive, and they can be difficult to test manually. Testing frameworks can help frontend engineers to automate their tests and to catch bugs early on before they cause problems for users.
There are different testing frameworks available, and the best one for you will depend on what your specific needs and preferences are. In this article, we will discuss some of the most popular testing frameworks.
Testing Frameworks for Frontend Engineers
Jest:
Jest is a JavaScript testing framework developed by Facebook. It is a pretty popular
choice for frontend engineers because it is easy to use and has a wide range of features.
Jest can be used to test unit, integration, and end-to-end (E2E) tests.
test('adds 1 + 2 to equal 3', () => {
expect(1 + 2).toBe(3);
});
Mocha:
Mocha is another popular JavaScript testing framework. It is similar to Jest, but
it has a few different features. Mocha is known for its flexibility and its ability
to integrate with other testing frameworks.
const assert = require('chai').assert;
describe('Math', function() {
it('should return 3 when adding 1 and 2', function() {
assert.equal(1 + 2, 3);
});
});
Jasmine:
Jasmine is a JavaScript testing framework that is based on behaviour-driven development
(BDD). BDD is a testing approach that focuses on the behaviour of the code being
tested. Jasmine is a good choice for frontend engineers who want to write tests that
are easy to read and understand.
describe('Math', () => {
it('should return 3 when adding 1 and 2', () => {
expect(1 + 2).toEqual(3);
});
});
Karma:
Karma is a test runner that can be used with a variety of testing frameworks, including
Jest, Mocha, and Jasmine. Karma is a good choice for frontend engineers who want
to run their tests in a continuous integration (CI) environment.
describe('Math', () => {
it('should return 3 when adding 1 and 2', () => {
expect(1 + 2).toBe(3);
});
});
Karma is a test runner that can be used with a variety of testing frameworks, including Jest, Mocha, and Jasmine.
Protractor:
Protractor is a testing framework that is specifically designed for Angular applications.
Protractor uses Selenium WebDriver to automate the testing of Angular applications.
describe('Angular App', () => {
it('should display welcome message', () => {
browser.get('/');
expect(element(by.css('app-root h1')).getText()).toEqual('Welcome to my app!');
});
});
Cypress:
Cypress is a modern end-to-end testing framework designed for web applications. It offers features like real-time reloading, automatic waiting, and integrated testing to make writing and maintaining tests easier and more efficient for frontend engineers.
describe('Math', () => {
it('should return 3 when adding 1 and 2', () => {
cy.visit('/');
cy.get('.math-operation').click();
cy.get('.result').should('have.text', '3');
});
});
Choosing a Testing Framework
The best way to choose a testing framework is to try out a few different ones and see which one you like the best. When choosing a testing framework, there are a few things to keep in mind:
- The features that the framework offers.
- The ease of use of the framework.
- The documentation for the framework.
- The community support for the framework.
- The compatibility of the framework with your development environment.
Writing Tests
Once you have chosen a testing framework, you can start writing tests for your frontend applications. There are a few different types of tests that you can write:
- Unit tests: Unit tests test individual units of code, such as functions or classes.
// Jest
function add(a, b) {
return a + b;
}
test('Add function adds two numbers', () => {
expect(add(1, 2)).toBe(3);
});
- Integration tests: Integration tests test how different units of code interact with each other.
// Jest
function multiply(a, b) {
return a * b;
}
function addAndMultiply(a, b, c) {
const sum = add(a, b);
return multiply(sum, c);
}
test('Add and Multiply functions work together', () => {
expect(addAndMultiply(1, 2, 3)).toBe(9);
});
- End-to-end (E2E) tests: E2E tests test the entire application from start to finish.
// Cypress
describe('E2E Test Example', () => {
it('Visits the website and performs an action', () => {
cy.visit('https://example.com');
cy.get('input[name="search"]').type('E2E testing{enter}');
cy.get('.search-results').should('contain', 'E2E testing');
});
});
When writing tests, it is important to follow good testing practices. These practices will help you to write tests that are reliable and maintainable.
Running Tests
Once you have written your tests, you need to run them. You can run your tests manually, or you can use a continuous integration (CI) server. A CI server will run your tests automatically every time you make a change to your code.
Testing is an important part of the development process, and it is especially important for frontend engineers. Testing frameworks can help frontend engineers to automate their tests and to catch bugs early on, before they cause problems for your users.